How to List and Print All Layers in PyTorch Model
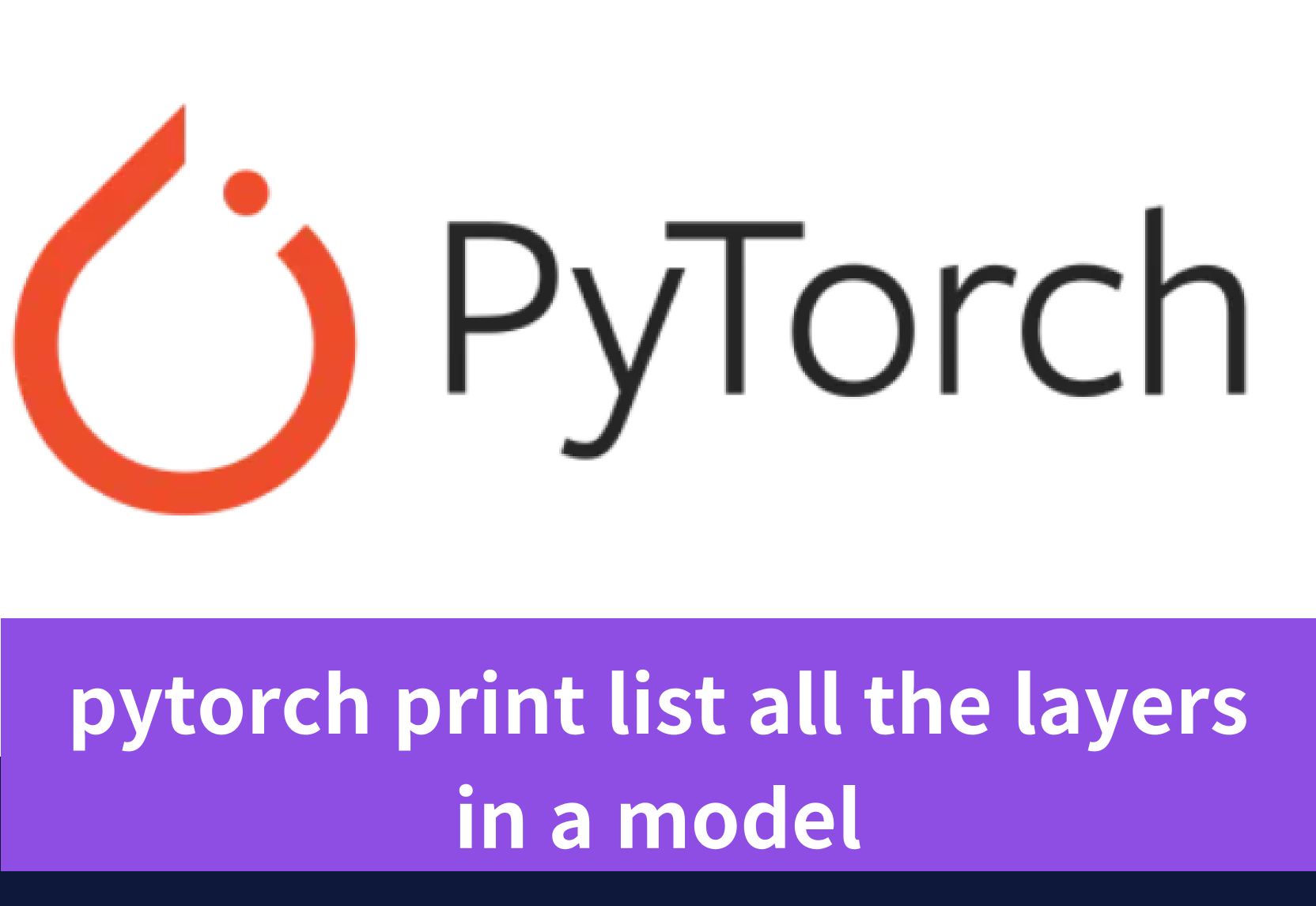
Introduction
In PyTorch, a well-liked tool for deep learning, you might find yourself needing to see and list every layer of your model. This is pretty handy when you’re trying to get how the model is put together, looking at its parts, or working with certain layers directly. This blog post will walk through three different ways you can go about listing and showing all the layers in your pytorch model. Moreover, this blog is going to introduce an excellent way for you to run Pytorch — on a GPU Cloud.
Understanding PyTorch Models
Inside a pytorch model, there are different layers that each do their own thing to the data they get. These layers work together to shape up what’s known as the architecture of your model. During training time, certain values within these layers adjust themselves — these are called trainable parameters — aiming to make your model smarter over time.
The Basics of PyTorch Model Architecture
In PyTorch, when you’re setting up a model, what you do is extend the nn.Module
class to create your own structure. Inside this setup, there's a must-have method called forward
. This one is crucial because it takes in your data and then marches it through the layers of your model one by one until it spits out the result. Think of each layer as its own little worker, like nn.Linear
for straight-up connections or nn.Conv2d
for filtering images.
To make sure these workers line up correctly and pass things along in order, we use something called the nn.Sequential
class. With this handy tool, all you have to do is list out your layers in order they should work on the data. It’s like telling them: "You first! Now you go!" And so on until every piece has done its job.
Key Components of PyTorch Models
In PyTorch, there are a few important parts that really matter. For starters, we’ve got what’s called a pretrained model. This is basically a ready-to-go model that has already learned a lot from being trained on tons of data. It knows certain things because it remembers the weights and biases from its training time. When we use one of these pretrained models, we’re taking all that knowledge it picked up and applying it to whatever task we have at hand.
Setting Up Your Environment for PyTorch
Before diving into the cool stuff we’re going to talk about later in this blog, you’ve got to get your computer ready. This means putting a few things on it like Python, PyTorch, and torchvision.
First off, Python is what you’ll be using when you do deep learning with PyTorch. Make sure it’s set up on your computer. If not, head over to the official Python website where you can grab and install it.
After that comes installing PyTorch itself. It’s basically the star of the show for deep learning in PyTorch land. It gives you all sorts of tools and goodies for making and teaching neural networks how to learn stuff by themselves. To get it onto your system, just use pip package manager with pip install torch
.
But wait — there’s more! You also need torchvision in your toolkit because if working with images is what excites you then this library has got some treats for ya’. Think pre-made datasets or models which are super handy for vision tasks plus ways to tweak those images so they’re just right for training purposes; again pip makes life easy here: pip install torchvision
.
Installing PyTorch
To get PyTorch on your computer, just open up where you type commands (like the terminal or command prompt) and put in:
pip install torch
This tells your computer to grab the newest version of PyTorch and set it up for you. You’ll need a good internet connection because this might take a bit of time.
Verifying PyTorch Installation
To make sure PyTorch is set up right, you can try out a basic Python script or use it in an interactive setting like Jupyter Notebook or the Python REPL. Just go into the Python environment you like best and bring in the torch module with this line of code:
import torch
Listing Layers in a PyTorch Model
Now that we’ve got the basics down about how a PyTorch model is put together, let’s dive into how we can see and list every layer it has.
Using .children() and .modules() Methods
To show every layer in a pytorch model, we can use the .children()
and .modules()
methods together with dot notation. Here's how you do it:
for name, module in model.named_modules():
print(name, module)
With this code snippet above, we’re using the named_modules()
method to get each part of our PyTorch model along with its name. By going through these one by one and showing their names and what they are, we manage to display all parts of our PyTorch setup.
What comes out from running this bit of Python is a list that tells us the names of different layers and shows us their modules too. This info is pretty handy for when you want to dive deeper into certain layers or change them up somehow.
Practical Examples of Listing Layers
When working with a PyTorch model, it’s really handy to know how to show and list all its parts. This can help you understand the model better, tweak certain bits of it, or fix issues. Here are some easy ways to do that:
- To get straight to a specific part using its name:
- You just use dot notation like this:
model.layer1.0.relu
- When you want to go through each part one by one and maybe change things as you go:
- A simple for loop lets you walk through named modules so you can work on them individually.
- If your goal is just seeing what parts there are along with their details:
- Combining the
named_modules()
method withprint
gives you both names and info about each module.
Printing Layers Details
Besides just naming the different parts of a PyTorch model, you might also want to share more about each part. This includes how many settings it has and what its results look like.
Accessing Specific Layer Information
If you’re diving into a PyTorch model and want to peek at the details of its layers, there’s a handy tool called “named_parameters” that can help. This function is like a treasure map; it guides you through all the named parameters in your model. By using this map, you can find specific information about each layer.
Here’s how it works: with just a few lines of code, you can print out everything about each layer.
for name, parameter in model.named_parameters():
print(name, parameter)
In these lines of code, name
tells us which layer we're looking at while parameter
shows us the tensor for that layer. It's like getting an X-ray view of your pytorch model - seeing what makes up each part without having to cut anything open!
On top of that, if you’re feeling adventurous or need something more user-friendly for complex tasks or deep analysis on those layers? Check out the ptrblck
library. It’s designed specifically for working smoothly with tensors and parameters in PyTorch models making things easier when dealing with complicated structures or needing detailed insights into how everything fits together.
Custom Functions to Print Layers
If you want to see certain layers or groups of them in a PyTorch model, you can whip up your own functions with the help of PyTorch and Python. These bits of code will need your model as an input and then they’ll show you just the layers you’re curious about.
Take this for instance: if you’re interested in checking out all the convolutional layers a model has, here’s how you could do it:
def print_conv_layers(model):
for name, module in model.named_modules():
if isinstance(module, nn.Conv2d):
print(name, module)
With this function, we go through each named module within our pytorch model and check whether it’s a nn.Conv2d
type. If that checks out, we get to see both its name and what’s inside.
Advanced Techniques
Besides the simple ways of looking at and printing parts of a PyTorch model, there are some cooler tricks you can do for a deeper dive.
Iterating Through Named Modules
When you’re working with a PyTorch model, going through named modules one by one lets you do certain things to each part. You can use the named_modules
function for this. It gives you an iterator that goes over every named module in your model.
With this approach, getting into and changing specific parts of the model becomes possible. For instance, if there’s a particular layer you want to tweak or check out its output for more analysis.
A handy way to keep track of these modules is by putting them into a dictionary. This makes it super easy to find and work on any module just by knowing its name. Here’s how it looks:
named_modules_dict = dict(model.named_modules())
conv1 = named_module_dict['conv1']
In the example above, conv1
points to the module called 'conv1'. Now that you've got hold of it directly from your list, doing whatever operation needed on it becomes straightforward.
Using Hooks to Extract Layers’ Output
In PyTorch, hooks let you set up special functions that get called at certain times when your model is running. Think of it like setting a checkpoint in a video game where something specific happens. These are really handy if you want to see what’s going on inside certain parts of your model.
To make these work, first off, you’ve got to write a function. This isn’t just any function though; it needs four pieces: module, input, output, and context. The most important bit here is the ‘output’ part because this lets you peek into what the layer’s spitting out and change things around if needed.
Here’s how you can whip up one of these hook functions:
def hook_fn(module,input,output):
# Do whatever with the output
print(output)
After crafting your function, attaching it to a layer in your model comes next. You do this by calling register_forward_hook
on whichever layer catches your fancy:
layer.register_forward_hook(hook_fn)
With everything set up now whenever that particular piece of code runs during the forward pass — bam! Your little custom function jumps into action letting you mess with or simply observe the outputs right then and there.
Accelerating PyTorch with Novita AI GPU Instance
Novita AI GPU Instance, a cloud-based solution, stands out as an exemplary service in this domain. It offers access to NVIDIA RTX 3090 GPUs, which are renowned for their ability to handle intensive computational workloads. This is particularly beneficial for PyTorch users who require the additional computational power that GPUs provide without the need to invest in local hardware.
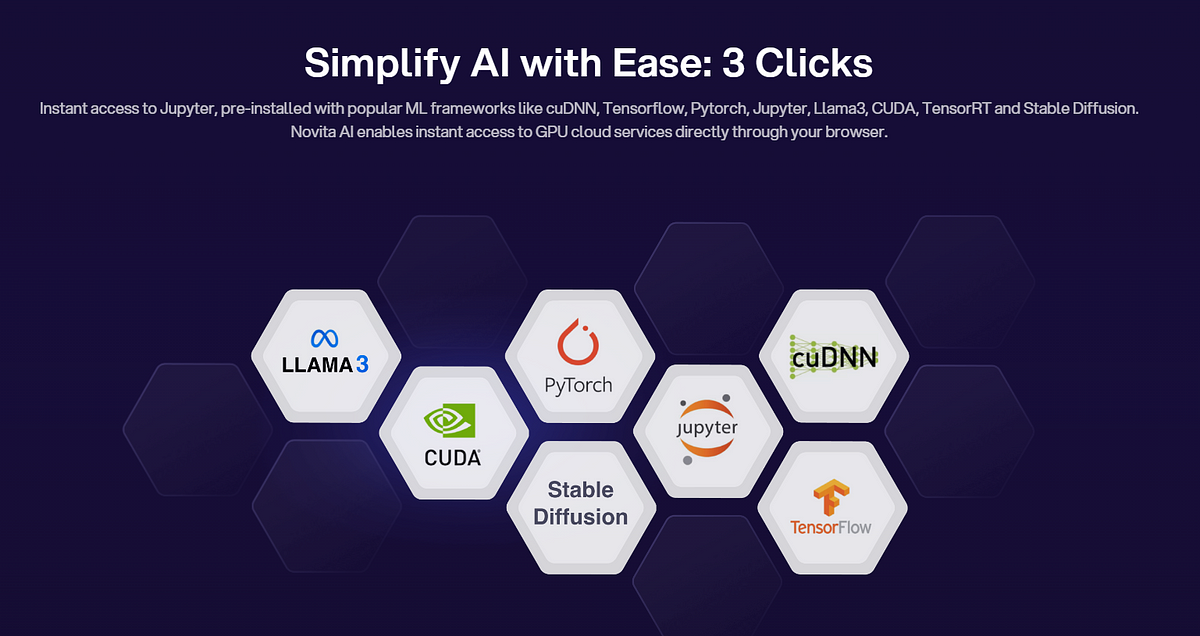
Here’s how Novita AI GPU Instance can be integrated with PyTorch to improve efficiency:
Computational Acceleration: PyTorch’s seamless integration with CUDA-enabled GPUs like the RTX 3090 provided by Novita AI GPU Instance allows for accelerated model training and inference. PyTorch’s .to(device)
method ensures that models and tensors are efficiently transferred to the GPU, unlocking the parallel processing capabilities necessary for deep learning.
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
Elastic Scalability: Novita AI GPU Instance offers the flexibility to scale resources up or down based on the project’s demands. This means that during periods of high computational need, additional GPU resources can be allocated, and once the task is complete, these resources can be deallocated, optimizing costs.
Cost Efficiency: The pay-as-you-go pricing model of cloud services like Novita AI GPU Instance ensures that users only pay for the resources they consume. This is particularly cost-effective for projects with fluctuating computational needs or for those requiring a burst of computational power for a limited time.
Conclusion
Getting to know the different parts of a PyTorch model is really important if you want to analyze and tweak your models well. By using tools like .children()
and .modules()
, it's pretty straightforward to list out and print every layer, which helps you understand how the model is put together. If you need more specific details about each layer, creating custom functions can do the trick for deeper analysis. Getting good at these methods means you'll be better at working with PyTorch models, making your deep learning projects even cooler.
Novita AI is the All-in-one cloud platform that empowers your AI ambitions. Integrated APIs, serverless, GPU Instance - the cost-effective tools you need. Eliminate infrastructure, start free, and make your AI vision a reality.
Recommended Reading: